Error: dynamodb table did not stabilize
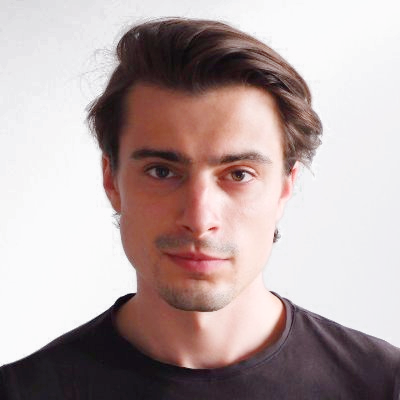
Answered by Rafal Wilinski
What's Causing This Error
This error message indicates that the table is not yet stable and ready to accept read or write operations.
For example, this can happen when a table is being created or updated, and DynamoDB is still allocating resources.
Solution: Here's How To Resolve It
To solve this error, you need to wait for the table to stabilize before attempting to perform any read or write operations. The time it takes for a table to stabilize depends on the size of the table and the number of throughput adjustments. You can check the table's status by calling the describe_table method and checking the TableStatus property.
Here is an example of how to check the status of a table:
import boto3 # Instantiate a client dynamodb = boto3.client('dynamodb', region_name='us-west-2') # Define the parameters table_name = "my_table" projection_expression = "attribute1, attribute2" # Perform the operation response = dynamodb.scan(TableName=table_name, ProjectionExpression=projection_expression) print(response)
Another way to solve this issue is to use the wait_until_table_exists
or wait_until_table_not_exists
method, polling the table's status until it reaches the desired state.
import boto3 # Instantiate a client dynamodb = boto3.client('dynamodb', region_name='us-west-2') # Define the parameters table_name = "my_table" # Perform the operation dynamodb.wait_until_table_exists(TableName=table_name)
It is also essential to keep in mind that increasing a table's read/write capacity may take a while for the changes to propagate and for the table to be fully available.
Other Common DynamoDB Errors (with Solutions)
- DynamoDB GetItem no item
- dynamodb property projection cannot be empty
- dynamodb stream missing fields
- DynamoDB ConditionExpression not working
- DynamoDB local error unable to access JAR file dynamodblocal.jar
- dynamodb table not exists
- dynamodb throttle error code
- does not support attribute type arn aws dynamodb
- dynamodb condition does not exist
- dynamodb local unable to open database file
- DynamoDB type item is not supported
- DynamoDB BatchSave not working
- DynamoDB service unavailable
- DynamoDB validation error
- dynamodb-admin is not recognized as an internal or external command