DynamoDB with AWS SAM - The Ultimate Guide
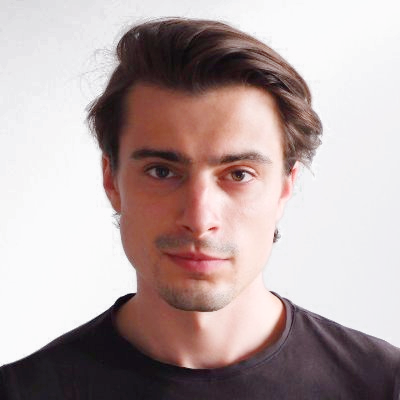
Written by Rafal Wilinski
Published on May 8th, 2020
Time to 10x your DynamoDB productivity with Dynobase [learn more]
Why DynamoDB with AWS SAM?
AWS Serverless Application Model (SAM) + DynamoDB work together really well. AWS maintains both pieces of them and each of them are "serverless". SAM has a list of special resources and property types which enable much faster development. Moreover, SAM integrates perfectly with other AWS services and has the best practices built-in.
In this tutorial, I'll show you how to build a simple API powered by SAM that uses DynamoDB as the data layer.
Step 1 - Prerequisites
Make sure you have the following installed:
- Docker
- AWS profile set up
- Node.js, preferably version > 10
- AWS SAM CLI
You should be able to run the following commands without any issues:
Step 2 - Create new AWS SAM project
Use the following command:
It will guide you through a short project setup wizard. Go ahead an choose:
1
- AWS Quick Start Templates1
- nodejs12.x runtime
And type your project name. After that, change the directory to the newly created one, and to make sure everything was set up correctly, use sam build
command.
Step 3 - The DynamoDB Table - our data store
First, we'll focus on adding the DynamoDB table into our application.
There are two ways to create a DynamoDB Table in SAM. The first one is the classical one, using AWS::DynamoDB::Table resource. This is the more advanced one but requires a complicated structure. Fortunately, our DynamoDB Table Designer might help you with that.
Second option, is to use simplified form of AWS::Serverless::SimpleTable resource. It supports only a single attribute primary key but is more intuitive:
Paste that into template.yaml
inside Resources
section.
Step 4 - Business Logic
Similar to the Serverless Framework tutorial, we'll create three functions for our CRUD app:
- Create Book
- Get Books
- Delete Book
Create function
After creating our SAM app, we should have a hello-world
directory in it. Rename it to books
and open app.js
file. Replace it with the following contents:
We will also need to change the HelloWorldFunction
part in template.yaml
file to the following one:
It does a series of things:
- Tells that the source code of our app is located inside
books
directory - Sets function handler to exported
create
function - Associates that function with API Gateway, specifically with
POST /book
endpoint
But that's not all. We need to give our Lambda function an IAM permission to manipulate our DynamoDB table. Luckily, AWS SAM has a set of special policy templates that make this process much faster. All we need to do is to include the following snippet into Properties
section of our function:
That was when it came to the create
function. We now want to have an ability to list everything that is in the database.
List/get many function
This one is easy. All we need to do is just run a Scan
operation against our table. Paste the create
function below:
Similar to the previous function, we need to add its definition to the template.yaml
file with slight modification:
We changed the handler from create
to list
, Event name to ListBooks
and method has changed to get
instead of post
.
Delete function
And for the last one, paste this code below the previous one:
The logic here is self-explanatory. Lambda is going to remove a record with the key name where it equals to the name
in path parameter. Don't forget about the template part:
Notice the curly braces in Path
. It will extract the part after /books/
into event.pathParameters.name
which is usable in code.
There's one more thing. In each of these functions, we're referring to variable TableName
, but right now, it is not set. We can fix that by setting it to the actual table name inside Globals:
Step 5 - Deployment
After changing the code, we need to build it again:
After that, there are two ways to test it: locally and by deploying it to the cloud. If you decide to test it locally with sam invoke local
, you might want to use the DynamoDB Local instance.
Go ahead and deploy it to the AWS using this command:
It will ask you a series of questions and save the answers inside samconfig.toml
so you won't be asked about them again.
After a while of waiting, it should output the URL of your API endpoint. Use it to fire a request to check if it works correctly. If it does, then congrats! You've just deployed a production-ready, cloud-native API with AWS Serverless Application Model and DynamoDB!