DynamoDBMapper Query Examples (DynamoDB Java Cheat Sheet)
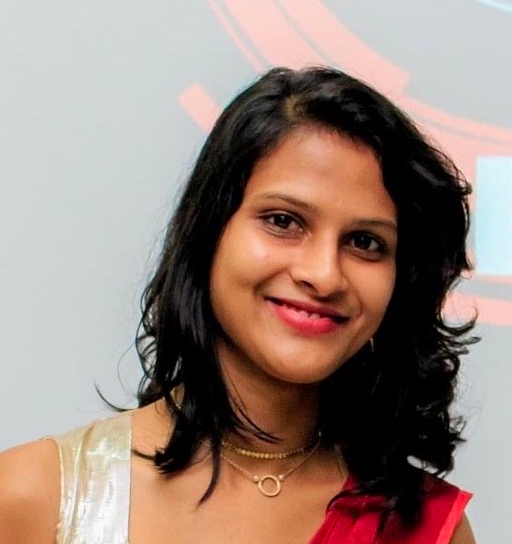
Written by Andrea Perera
Published on December 24th, 2021
- Setup
- Create a DynamoDB Table
- Connection
- Create a Model Class
- Put an Item
- Get an Item
- Update an Item
- Batch Put Item
- Batch Put Item & Delete
- Batch Get Items
- Scan with Filter
- Scan Parallel
- Query
- Delete an Item
- Batch Delete Items
- Delete a table
- Run DynamoDB Local
Time to 10x your DynamoDB productivity with Dynobase [learn more]
What is DynamoDBMapper?
DynamoDBMapper is a Java class for Amazon Web Services(AWS). This is the Java DynamoDB NoSQL Database SDK. With DynamoDBMapper, you can set up the relationship between elements in a DynamoDB database table and their related object instances using a domain class.
This cheat sheet is to guide you to set up your Java application, perform create, read, update, and delete (CRUD) operations and run queries using DynamoDBMapper Java class.
If you're looking for similar guide but for Node.js, you can find it here, for Rust, for Python and for Golang / Go here.
Table of Contents
- Setup
- Create Table
- Connection
- Creating a model class
- Put Item
- Get Item
- Update Item
- Batch Put Item
- Batch Put Item & Delete
- Batch Get Items
- Scan with Filter
- Scan Parallel
- Query
- Delete an Item
- Batch Delete Items
- Delete Table
- Run DynamoDB Local
Setup
Prerequisites
- Create a group/user and give AmazonDynamoDBFullAccess policy in IAM.
- Get the Access and Secret Key to configure the Java application.
- Create a Spring Boot Java application.
Note: make sure to install aws-java-SDK-dynamodb dependencies in your pom.xml file in the Java application.
Create a DynamoDB Table
To correctly persist the data, you need to create a table in DynamoDB.You can use AWS Console, Dynobase, AWS CLI, or use AWS-SDK for Java to create a table with a partition key (primary key).
Create a table named Product with ProductID as the partition key.
Note: You can create a table with a sort key (range key) which will be useful for Query. This allows you to retrieve a group of entries within a partition (having the same partition key).
Connection
- Add the Access, Secret Key, and region to store the DynamoDB table.
Create a Model Class
Let's create an object called Product which represents an item in a DynamoDB table. The Employee class is accessible via getter and setter methods to correctly persist the data using DynamoDBMapper.
- DynamoDBHashKey : is the Java annotation to map a property to a table attribute.
- DynamoDBHashKey: is the Java annotation for the hash key(partition key) which is the primary key.
- DynamoDBRangeKey: is the Java annotation for the range key(sort key)
Put an Item
To write a single item into the DynamoDB table. This will use the save method.
Get an Item
To get a single item from DynamoDB using Partition Key/hash key(ProductId) or the range key. This will use the load method.
Parameters for the load methods are
- class: The class to load, same as the DynamoDB table
- hashKey: The key of the object.
- rangeKey: The range key of the object(optional)
- config: The service call to fetch the object from DynamoDB is configured.
Update an Item
To update an item, 1st you will get the item that needs to be updated by the hash key/partition key(productId) and then you can update an item.
Batch Put Item
To write/put a batch of items into the DynamoDB table, you can use the batchSave method. The batchSave method of DynamoDBMapper is implemented using the BatchWriteItem API.
The below code is to write/put a batch of items into the Product DynamoDB table.
Batch Put Item & Delete
You can use BatchWriteItem to write or delete multiple items across multiple tables.
The below code is to write/put a batch of items into purchase and transaction tables and delete an item from the Product table. The primary key of the Purchase table consists of the PurchaseId and Quantity, PurchaseDate fields. The primary key of the ProductCategory table consists of the CategoryId and CategoryName fields.
It has a maximum write limit of 16MB and a maximum request limit of 25. Item updates are not possible with batch writes. In a batch, if there are two PutItem requests and one DeleteItem request, the failure of the PutItem request has no effect on the others.
Batch Get Items
To get a set of items from DynamoDB using Partition Key/hash key(ProductId) from one or more tables. This will use the batchload method.
Scan with Filter
You can use the scan method to scan through the entire table by using the FilterExpression operation you can filter out the results. To filter the results you can not use the partition or sort key.
Note: Scan performs better with small tables with fewer filters.
Scan Parallel
The parallel scan will be splitting tables into parts logically then "workers" scan segments in parallel. This is the quick fix if you want to scan larger amounts of tables. You can define the number of workers/Segments.
The below code is to scan parallel in the Purchase table.
Query
You should use a query operation to get a lookup to a certain partition. To query the table there should be a sort key along with the partition key (primary key). The query is quicker and cheaper than the scan.
The primary key of the ProductCategory table consists of the CategoryId and CategoryName(sort key). You can fetch up to 1MB of records.
Delete an Item
Deleting a single item from the DynamoDB table. You can use the delete method to delete an item.
Batch Delete Items
To delete a batch of items from one or more DynamoDB tables. You can use the batchDelete method.
Delete a table
To delete a table, go to the AWS console and select Tables from the navigation pane, then from the table list, select the table you want to delete.
You can use the code below to delete a table.
Run DynamoDB Local
If you need to use DynamoDB offline locally, you can use DynamoDB locally distributed by AWS or DynamoDB from localstack
First, install version 6. x or higher of the Java Runtime Environment (JRE) and obtain DynamoDB from here.
After that, extract the archive and copy its contents to a location Finally, open a terminal in the location where you extracted the files and run the below command.