AWS DynamoDB DocumentClient & Node.js - Complete Cheat Sheet
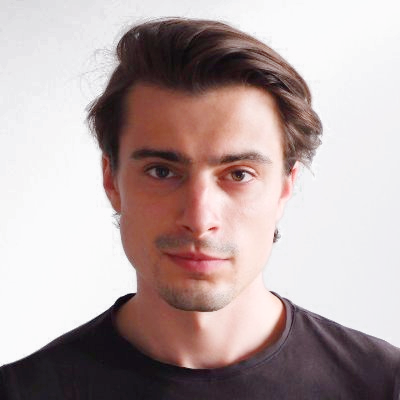
Written by Rafal Wilinski
Published on March 29th, 2020
- Setup
- Create table
- Delete table
- List Tables
- Get All Items / Scan in DynamoDB
- Get Item
- Batch Get Item
- Put Item aka Write
- Batch Write / Put Item
- Query for a Set of Items
- Query an Index
- Simple Transaction
- Read Transaction
- Query with Sorting
- Query (and Scan) DynamoDB Pagination
- Update Item
- Conditionally Update Item
- Increment Item Attribute
- Delete Item
- Delete All Items
- Run DynamoDB Local
Time to 10x your DynamoDB productivity with Dynobase [learn more]
DynamoDB Node.js Query Examples
This cheat sheet should help you understand how to perform a variety of operations starting from simple queries ending with complex transactions using AWS DynamoDB DocumentClient and Node.js.
There are two basic ways to interact with DynamoDB tables from Node.js applications:
- Class
AWS.DynamoDB
from AWS SDK for JavaScript/Typescript AWS.DynamoDB.DocumentClient
which simplifies working with DynamoDB items by abstracting away DynamoDB Types and converting responses to native JS
This cheat sheet will mostly focus on DocumentClient
but some of the operations like creating tables must be run using classical DynamoDB service.
If you're looking for similar cheat sheet but for Python, you can find it here, for Java, for Golang / Go here, and for Rust.
Bonus: AWS recently announced Node.js AWS SDK v3. I wrote a tutorial on how to use new SDK V3, especially in the DynamoDB context
DynamoDB is hard. We get it.
Dynobase's codegen figures out queries and writes code for you!
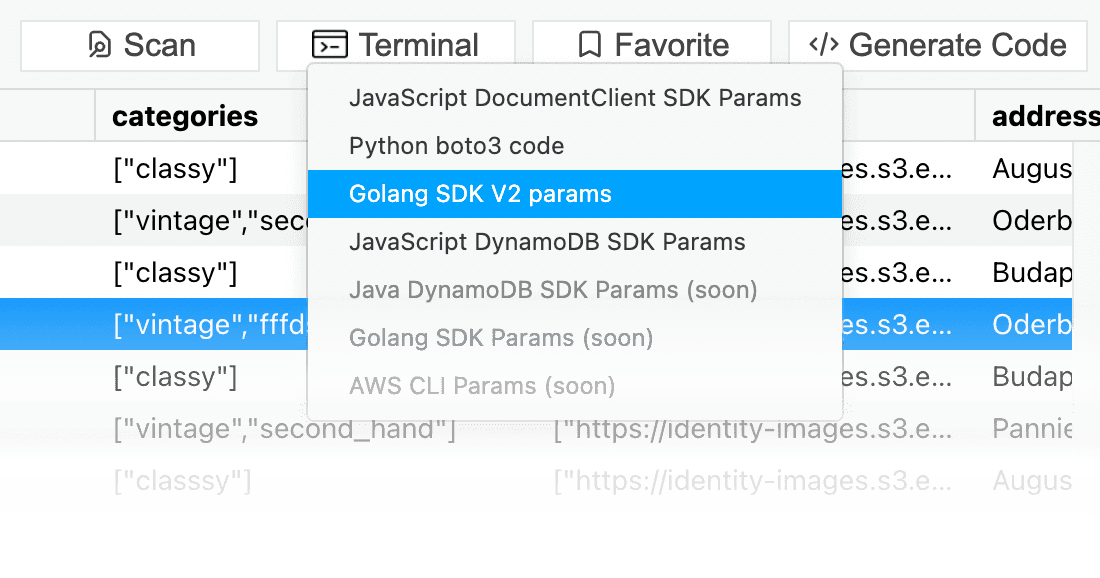
Table of Contents
- Setting up
- Create Table
- Delete Table
- List Tables
- Get All Items / Scan
- Get Item
- Batch Get Item
- Put Item
- Batch Write Item
- Query for a Set of Items
- Query an Index
- Update Item
- Conditionally Update Item
- Increment Item Attribute
- Delete Item
- Delete All Items
- Simple Transaction
- Read Transaction
- Query with Sorting
- Query Pagination
- Run DynamoDB Local
Setup
Setting up your Node.js application to work with DynamoDB is fairly easy. First, make sure that aws-sdk
is installed, either by running yarn add aws-sdk
or npm i aws-sdk --save
. Then, paste following piece of code:
As one of the Node.js best practices is to avoid callback hell, we'll be ending up all of our SDK calls with .promise()
call in order to get Promises returned from SDK. It will also allow us to use async/await
syntax which makes our programs much more readable.
Keep in mind that using access and secret keys is against best security practices, and you should instead use IAM roles/policies to interact with DynamoDB. This code, if ran on Lamba function or EC2 instance, will automatically use IAM Role attached to it.
Create table
DynamoDB structures data in tables, so if you want to save some data to DynamoDB, first you need to create a table. You can do that using AWS Console, AWS CLI or using AWS-SDK for Javascript, like this:
After this call resolves, it does not necessarily mean that table status is ACTIVE
and it's is ready for read and write operations. Before start manipulating items in it, we should check if it's in ACTIVE
state first using describeTable
function ran every 5 seconds until it is ready:
Delete table
If you changed your mind and need to remove DynamoDB table, don't worry, it's simple:
Keep in mind that Dynobase is capable to removing tables too.
List Tables
If you want to check what tables are available at your disposal in current region, use listTables
call. Keep in mind that if selected region has more than 100 tables you'll have to paginate through them to fetch a complete list.
Get All Items / Scan in DynamoDB
After our table is provisioned and it's in ACTIVE
state, first thing that we probably would like to do is get all items in it aka use (DynamoDB Scan operation):
If you want to narrow your search results, use FilterExpressions
combined with ExpressionAttributeNames
object like so:
You can find full reference how to write FilterExpression
s in this post by Alex Debrie.
The snippet above will in fact return all the items in the table under one condition - you have less than 1MB of data inside it. If your table is bigger than that, you'll have to run Scan command a few times in a loop using pagination.
Get Item
If you know the exact Partition Key (and Sort Key if using composite key) of the item that you want to retrieve from the DynamoDB table, you can use get
operation:
Batch Get Item
DocumentClient
is also capable of running bunch of get
operations in a single call to the DynamoDB service:
As you can see, the RequestItems
objects can accept multiple table names and can fetch multiple items from multiple tables in a single call. Keep in mind that number of items retrieved using batchGet
is limited to 100 items or 16MB of data.
Moreover, if you exceed table capacity, this call will return UnprocessedKeys
attribute containing a map of keys which weren't fetched.
Put Item aka Write
put
operation creates a new item, or replaces an old item with a new item if it's using the same key(s):
Batch Write / Put Item
If you need to insert, update or delete multiple items in a single API call, use batchWrite
operation. It bundles multiple database requests against multiple tables into a single SDK call. It decreases amount of network calls needed to be made, reduces the overall latency and makes your application faster.
Example request deleting one item with key id = 123
and putting another to the same table:
If you want to write into multiple tables at once, simply specify these tables inside RequestItems
object like this:
If you are curious about performance of batchWrite
, head to dynamodb-performance-testing repo by Alex DeBrie.
Query for a Set of Items
If your table has composite key (which is the best practice), in order to get a collection of items sharing the same Parition Key, use Query
method. It also allows to use multiple operators for SortKey such as begins_with
or mathematical ones like >
, =
, >=
and so on.
Keep in mind that Query
can return up to 1MB of data and you can also use FilterExpression
s here to narrow the results on non-key attributes.
If you don't know how to construct your Query and its attributes, head to our DynamoDB Query Builder which will generate code for you. You can also use Query Code Generation feature inside Dynobase.
Tired of AWS Console? Try Dynobase.
First 7 days are. No credit card needed.
Query an Index
DynamoDB allows querying not only on the main table index, but also on LSIs (Local Secondary Indexes) and GSIs (Global Secondary Indexes).
To do that in SDK, you need to change two things:
- Specify the index you want to query using
IndexName
parameter - Provide correct
KeyConditionExpression
with correspondingExpressionAttributeValues
and ExpressionAttributeNames`
So, to give you an example. Imagine your table is having a Global Secondary Index called GSI1
with attributes gsi1pk
and gsi1sk
. If you'd like to query that index in Node.js, it will look like this:
Simple Transaction
DynamoDB also support transactions - they allow to run multiple write operations atomically meaning that either all of operations are executed succesfully or none of them. It is especially useful when dealing with applications where data integrity is essential, e.g. in e-commerce - adding an item to a cart and decrementing count of items still available to buy.
Such flow should:
- Should happen atomically - these two operations should be treated as one, we don't want to have a single moment in time where there's a discrepancy in items count
- Should succeed only if count of items available to buy is greated than zero
Described flow can be modelled in Javascript & DocumentClient like this:
If you want to learn more about transactions, head to our DynamoDB Transactions Guide.
Read Transaction
Transactions can be also used for reading data atomically. Like in batchGet
, you can retrieve the data from multiple tables in a single call:
If you want to learn more about transactions, head to our DynamoDB Transactions Guide.
Query with Sorting
Unfortunately, DynamoDB offers only one way of sorting the results on the database side - using the sort key. If your table does not have one, your sorting capabilities are limited to sorting items in application code after fetching the results. However, if you need to sort DynamoDB results on sort key descending or ascending, you can use following syntax:
Query (and Scan) DynamoDB Pagination
Both Query and Scan operations return results with up to 1MB of items. If you need to fetch more records, you need to invoke a second call to fetch the next page of results. If LastEvaluatedKey
is present in response object, this table has more items like requested and another call with ExclusiveStartKey
should be sent to fetch more of them:
Learn more about Pagination in DynamoDB.
Update Item
DynamoDB update
operation in Node.js consists of two main parts:
- Part which item to update (
Key
), similar to get - Part what in the selected item should be updated (
UpdateExpression
andExpressionAttributeValues
)
Conditionally Update Item
Sometimes we want to update our record only if some condition is met, e.g. item is not soft-deleted (does not have deletedAt
attribute set). To do that, use ConditionExpression
which has similar syntax to the FilterExpression
:
In this example the name
attribute of the record with partition key id = 123
in table my-table
will be only updated if this item does not have attribute deletedAt
and its attribute company
has value Apple
.
Increment Item Attribute
Incrementing a Number value in DynamoDB item can be achieved in two ways:
- Get item, update the value in the application code and send a
put
request back to DDB overwriting item - Using
update
operation
While it might be tempting to use first method because Update syntax is unfriendly, I strongly recommend using second one because of the fact it's much faster (requires only one request) and atomic:
In this example the score
attribute of the record with partition key id = 123
in table my-table
will incremented by one. Of course, you can use other mathematical operators too.
Delete Item
Removing single item from table is very similar to Get Item operation. The parameters of the call are actually exactly the same, the only difference is that we call delete
instead of get
:
Delete All Items
Unfortunately, there's no easy way to delete all items from DynamoDB just like in SQL-based databases by using DELETE FROM my-table;
. To achieve the same result in DynamoDB, you need to query/scan to get all the items in a table using pagination until all items are scanned and then perform delete
operation one-by-one on each record.
If you don't want to run this script on your own (it might be really time consuming), truncating table is possible just with few clicks using Dynobase.
Run DynamoDB Local
If you need to use DynamoDB offline locally, you can use DynamoDB local distributed by AWS or DynamoDB from Localstack. Connecting to it is as easy as changing the endpoint
parameter DynamoDB
or DocumentClient
constructor:
If you want to see your local tables and data in them, you can use Dynobase to query and modify items in offline tables.
Learn more about running DynamoDB locally.
Bonus!
If you're looking for Serverless Framework based CRUD project which uses other AWS Lambda and DynamoDB best practices with Node.js, you can find one on our Github.