Query Index in DynamoDB Using Java (Guide w/ Code Examples)
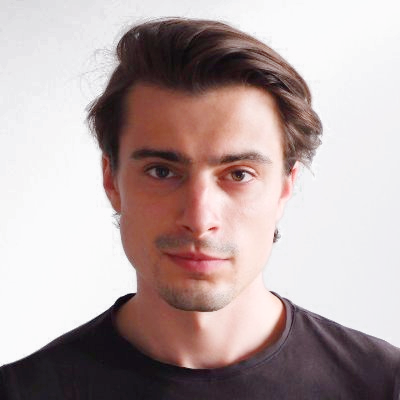
Provided by Rafal Wilinski
You can use the QueryRequest
class offered in the DynamoDB SDK to perform a query. You will have to provide a Table Name, Optional Index Name, and a Key Condition Expression. If you've provided an Index Name, DynamoDB will query the index belonging to the table. However, if the index is not found, DynamoDB will throw an error.
The following code example queries the "MyTable" table using the LastNameIndex
index for items where the lastName
attribute is "Smith". The QueryRequest
class is used to specify the index name and the key condition expression, which in this case, is lastName = :val
. The expressionAttributeValues
map is used to specify the value to be used in the key condition expression, in this case ":val
" is mapped to the value Smith
.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.AttributeValue; import com.amazonaws.services.dynamodbv2.model.QueryRequest; import com.amazonaws.services.dynamodbv2.model.QueryResult; public class QueryIndexExample { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); Map<String, AttributeValue> expressionAttributeValues = new HashMap<>(); expressionAttributeValues.put(":val", new AttributeValue().withS("Smith")); QueryRequest queryRequest = new QueryRequest() .withTableName("MyTable") .withIndexName("LastNameIndex") .withKeyConditionExpression("lastName = :val") .withExpressionAttributeValues(expressionAttributeValues); QueryResult result = client.query(queryRequest); result.getItems().forEach(item -> System.out.println(item)); } }
Similar Code Examples
- Batch Write Using DynamoDB Mapper
- GetItem in DynamoDB Using Java
- Update Item in DynamoDB Using Java
- Delete Table in DynamoDB Using Java
- Get All Items from DynamoDB Using Java
- Batch Load Using DynamoDB Mapper
- Delete Expression in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Delete All Items in DynamoDB Using Java
- Update Expression in DynamoDB Using Java
- Batch Update Using DynamoDB Mapper
- Delete Multiple Items in DynamoDB Using Java
- Batch Query in DynamoDB Using Java
- Delete in DynamoDB Using Java
- Update Multiple Items in DynamoDB Using Java