Conditional Update in DynamoDB Using Java (Guide w/ Code Examples)
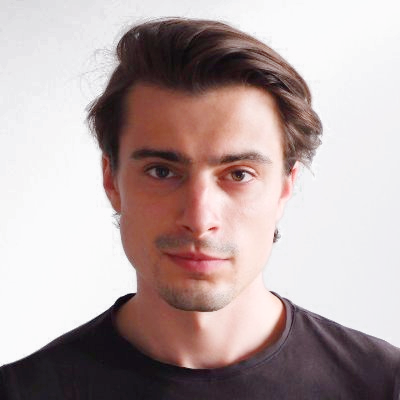
Provided by Rafal Wilinski
DynamoDB provides the ability to update an item conditionally based on certain conditions. You can use the updateItem
method with a condition expression in Java. In the below example, the updateItem
method is used to update an item in a table named "Product." The key of the item to update is specified using the key map, which maps attribute names to attribute values.
The updateExpression
parameter is used to specify the update to be performed on the item. The expressionAttributeValues
map provides the values for any placeholders in the update expression. The conditionExpression
parameter is used to specify a condition that must be true for the update to be performed. If the condition is not true, the update will be skipped, and no error will be returned.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.AttributeValue.AttributeValue; import com.amazonaws.services.dynamodbv2.model.AttributeValue.M; import com.amazonaws.services.dynamodbv2.model.UpdateItemRequest; import java.util.Map; public class ConditionalUpdateExample { public static void main(String[] args) { // Create a new client AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build(); // Define the key of the item to update Map<String, AttributeValue> key = new HashMap<String, AttributeValue>(); key.put("id", new AttributeValue().withS("123")); // Define the update expression String updateExpression = "SET price = :newPrice, quantity = :newQuantity"; // Define the values for the update expression Map<String, AttributeValue> expressionAttributeValues = new HashMap<String, AttributeValue>(); expressionAttributeValues.put(":newPrice", new AttributeValue().withN("999")); expressionAttributeValues.put(":newQuantity", new AttributeValue().withN("20")); // Define the condition expression String conditionExpression = "quantity < :oldQuantity"; // Define the values for the condition expression Map<String, AttributeValue> expressionAttributeValues = new HashMap<String, AttributeValue>(); expressionAttributeValues.put(":oldQuantity", new AttributeValue().withN("50")); // Create the update request UpdateItemRequest request = new UpdateItemRequest() .withTableName("Product") .withKey(key) .withUpdateExpression(updateExpression) .withExpressionAttributeValues(expressionAttributeValues) .withConditionExpression(conditionExpression); // Send the request to update the item client.updateItem(request); } }
Similar Code Examples
- Query Global Secondary Index in DynamoDB Using Java
- Query Index in DynamoDB Using Java
- Get All Items from DynamoDB Using Java
- Insert in DynamoDB Using Java
- Batch Put Item in DynamoDB Using Java
- Query Date Range in DynamoDB Using Java
- Update Multiple Attributes in DynamoDB Using Java
- Delete Multiple Items in DynamoDB Using Java
- Update Attribute in DynamoDB Using Java
- Batch Write Using DynamoDB Mapper
- Delete in DynamoDB Using Java
- Update Expression in DynamoDB Using Java
- Delete Table in DynamoDB Using Java
- Batch Write Item in DynamoDB Using Java
- Batch Insert in DynamoDB Using Java