Delete in DynamoDB Using Java (Guide w/ Code Examples)
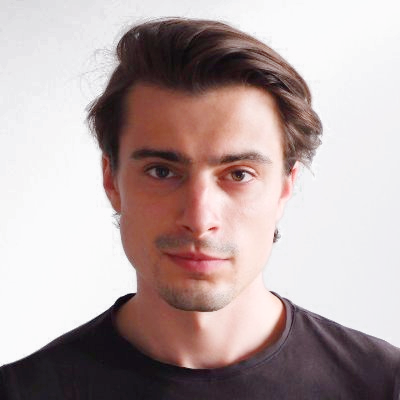
Provided by Rafal Wilinski
To delete an item from a DynamoDB table in Java, you can use the deleteItem
method of the AmazonDynamoDB
client.
In the snippet defined below, YourTableName
is the name of the table you want to delete the item from, YourHashKeyValue
is the value of the hash key for the item you want to delete, and YourRangeKeyValue
is the value of the range key for the item you want to delete.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.DeleteItemRequest; import com.amazonaws.services.dynamodbv2.model.DeleteItemResult; public class DynamoDBDeleteExample { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); DeleteItemRequest request = new DeleteItemRequest() .withTableName("YourTableName") .withKey( new Key().withHashKeyElement(new AttributeValue("YourHashKeyValue")) .withRangeKeyElement(new AttributeValue("YourRangeKeyValue"))); DeleteItemResult result = client.deleteItem(request); System.out.println("Item deleted successfully!"); } }
Similar Code Examples
- Batch Put Item in DynamoDB Using Java
- Get All Items from DynamoDB Using Java
- Remove with DynamoDB Mapper
- Batch Save Using DynamoDB Mapper
- Delete All Using DynamoDB Mapper
- Update Item in DynamoDB Using Java
- Delete Table in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Delete All Items in DynamoDB Using Java
- Get in DynamoDB Using Java
- Batch Delete Using DynamoDB Mapper
- Batch Get in DynamoDB Using Java
- Query Global Secondary Index in DynamoDB Using Java
- Query Local Secondary Index in DynamoDB Using Java
- Batch Load Using DynamoDB Mapper
Spend less time in the AWS console, use Dynobase.
Try 7-day free trial. No credit card needed.
Product Features
DynamoDB Tools
DynamoDB Info
© 2024 Dynobase