Batch Load Using DynamoDB Mapper (Guide w/ Code Examples)
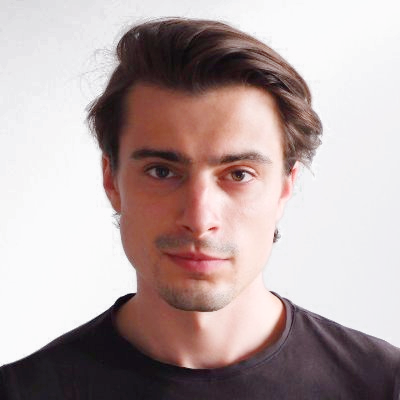
Provided by Rafal Wilinski
You can use the DynamoDB mapper's batchLoad
method to load multiple items from a DynamoDB table.
We first build a list of primary key values for the items we want to retrieve from the DynamoDB table. Then we create a DynamoDBQueryExpression
object and set the primary key values using the withKeys
method. We pass this query expression to the batchLoad
method along with the class of the item we are loading, and it will return the list of items that match the primary keys.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBMapper; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBQueryExpression; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class DynamoDBMapperBatchLoadExample { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); DynamoDBMapper mapper = new DynamoDBMapper(client); List<String> ids = Arrays.asList("1", "2", "3"); List<Object> keys = new ArrayList<Object>(); for (String id : ids) { Item item = new Item(); item.setId(id); keys.add(item); } DynamoDBQueryExpression<Item> queryExpression = new DynamoDBQueryExpression<Item>().withKeys(keys); List<Item> items = mapper.batchLoad(Item.class, keys); System.out.println("Items retrieved successfully!"); System.out.println(items); } }
Similar Code Examples
- Insert in DynamoDB Using Java
- Query Local Secondary Index in DynamoDB Using Java
- Batch Read in DynamoDB Using Java
- Delete Multiple Items in DynamoDB Using Java
- Conditional Update in DynamoDB Using Java
- Update Expression in DynamoDB Using Java
- Batch Put Item in DynamoDB Using Java
- Query Index in DynamoDB Using Java
- GetItem in DynamoDB Using Java
- Update Multiple Items in DynamoDB Using Java
- Query Date Range in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Delete by Hashkey Using DynamoDB Mapper
- Delete Table in DynamoDB Using Java
- Batch Write Using DynamoDB Mapper
Tired of AWS Console? Try Dynobase.
Try 7-day free trial. No credit card needed.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase