Query Global Secondary Index in DynamoDB Using Java (Guide w/ Code Examples)
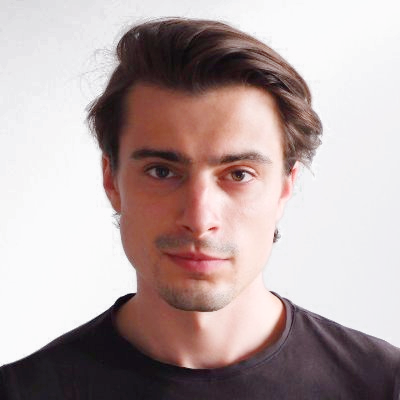
Provided by Rafal Wilinski
To query a global secondary index (GSI) in a DynamoDB table in Java, you can use the query
method of the AmazonDynamoDB
client by providing the GSI Name, Table Name, and Key Condition Expression.
In the below example, YourTableName
is the name of the table that contains the GSI you want to query, YourGSI
is the name of the GSI you want to query, GSIKeyAttribute
is the name of the key attribute on the GSI, and YourSearchValue
is the value you want to search for in the GSI.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.Attribute.Attributevalue; import com.amazonaws.services.dynamodbv2.model.queryrequest; import com.amazonaws.services.dynamodbv2.model.queryresult; public class DynamoDBQueryGSIExample { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); QueryRequest request = new QueryRequest() .withTableName("YourTableName") .withIndexName("YourGSI") .withKeyConditionExpression("GSIKeyAttribute = :value") .withExpressionAttributeValues( new AttributeValue().withS(":value", "YourSearchValue")); QueryResult result = client.query(request); System.out.println("Query successful!"); System.out.println(result); } }
Similar Code Examples
- Update Item in DynamoDB Using Java
- Remove with DynamoDB Mapper
- Batch Delete Using DynamoDB Mapper
- Update Multiple Items in DynamoDB Using Java
- Get Item Request in DynamoDB Using Java
- Get in DynamoDB Using Java
- Query Index in DynamoDB Using Java
- Delete Record in DynamoDB Using Java
- Get Multiple Items in DynamoDB Using Java
- Conditional Update in DynamoDB Using Java
- Remove Attribute in DynamoDB Using Java
- Batch Query in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Update Expression in DynamoDB Using Java
- Delete All Using DynamoDB Mapper
Tired of switching accounts and regions? Use Dynobase.
Try 7-day free trial. No strings attached.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase