Batch Save Using DynamoDB Mapper (Guide w/ Code Examples)
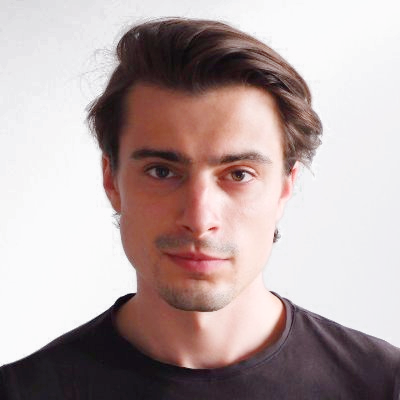
Provided by Rafal Wilinski
You can use the BatchWriteItemRequest
class to perform Batched Save operations on DynamoDB.
This example saves two items (of class Item
) to the table "my-table" in a single batch write operation. The DynamoDBMapper
's batchSave
method converts the objects into WriteRequest
objects and puts them into a Map
of request items. The BatchWriteItemRequest
object is then created using this map and passed to the batchWriteItem
method of the DynamoDB client to save the items in a single call.
import software.amazon.awssdk.services.dynamodb.DynamoDbClient; import software.amazon.awssdk.services.dynamodb.model.AttributeValue; import software.amazon.awssdk.services.dynamodb.model.PutItemRequest; import software.amazon.awssdk.services.dynamodb.model.WriteRequest; import software.amazon.awssdk.services.dynamodb.model.BatchWriteItemRequest; import software.amazon.awssdk.services.dynamodb.model.BatchWriteItemResponse; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBMapper; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBMapperConfig; import java.util.ArrayList; import java.util.List; import java.util.Map; public class BatchSaveExample { public static void main(String[] args) { DynamoDbClient ddb = DynamoDbClient.create(); DynamoDBMapper mapper = new DynamoDBMapper(ddb); String tableName = "my-table"; List<Object> itemsToSave = new ArrayList<>(); itemsToSave.add(new Item("12345", "value1", "value2")); itemsToSave.add(new Item("67890", "value3", "value4")); Map<String, List<WriteRequest>> requestItems = mapper.batchSave(itemsToSave); BatchWriteItemRequest batchWriteItemRequest = BatchWriteItemRequest.builder() .requestItems(requestItems) .build(); BatchWriteItemResponse response = ddb.batchWriteItem(batchWriteItemRequest); } } class Item { private String id; private String attribute1; private String attribute2; public Item() {} public Item(String id, String attribute1, String attribute2) { this.id = id; this.attribute1 = attribute1; this.attribute2 = attribute2; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getAttribute1() { return attribute1; } public void setAttribute1(String attribute1) { this.attribute1 = attribute1; } public String getAttribute2() { return attribute2; } public void setAttribute2(String attribute2) { this.attribute2 = attribute2; } }
Similar Code Examples
- Remove Item in DynamoDB Using Java
- Update Item in DynamoDB Using Java
- Batch Read in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Batch Get in DynamoDB Using Java
- Batch Put Item in DynamoDB Using Java
- Batch Query in DynamoDB Using Java
- Delete All Using DynamoDB Mapper
- Query Local Secondary Index in DynamoDB Using Java
- Batch Insert in DynamoDB Using Java
- Query Global Secondary Index in DynamoDB Using Java
- Update Attribute in DynamoDB Using Java
- Delete Multiple Items in DynamoDB Using Java
- Batch Delete Using DynamoDB Mapper
- Get Item Request in DynamoDB Using Java
Dynobase is a Professional GUI Client for DynamoDB
Try 7-day free trial. No credit card needed.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase