Delete Expression in DynamoDB Using Java (Guide w/ Code Examples)
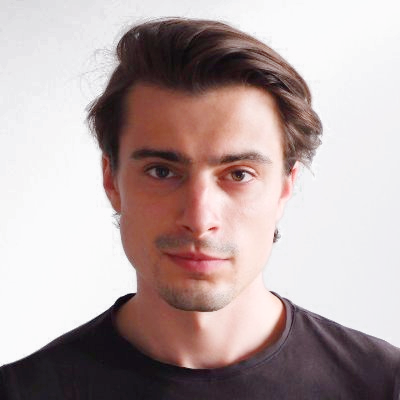
Provided by Rafal Wilinski
To delete an item from a DynamoDB table using a delete expression in the AWS SDK for Java, you can use the deleteItem
method of the DynamoDB
class. This method allows you to specify a DeleteExpression object in the DeleteItemRequest
to specify a delete condition for the item.
The following code shows how you could use the deleteItem
method with a delete expression to delete an item from a table named "myTable" only if the item has an attribute named "attr" with a value of "foo". If the delete condition is not met, the deleteItem
method will return.
import software.amazon.awssdk.services.dynamodb.DynamoDBClient; import software.amazon.awssdk.services.dynamodb.model.AttributeValue; import software.amazon.awssdk.services.dynamodb.model.DeleteItemRequest; import software.amazon.awssdk.services.dynamodb.model.DeleteItemResponse; import software.amazon.awssdk.services.dynamodb.model.DynamoDBException; import software.amazon.awssdk.services.dynamodb.model.Key; import software.amazon.awssdk.services.dynamodb.model.KeySchemaElement; import software.amazon.awssdk.services.dynamodb.model.KeyType; import software.amazon.awssdk.services.dynamodb.model.DeleteExpression; import java.util.HashMap; import java.util.Map; public class DeleteExpressionExample { public static void main(String[] args) { // Create a DynamoDB clientDynamoDBClient client = DynamoDBClient.create(); // The primary key of the item to deleteKey key = Key.builder() .partitionKey(KeySchemaElement.builder() .attributeName("id") .keyType(KeyType.HASH) .build()) .build(); // The delete condition for the item Map<String, AttributeValue> conditionExpressionAttributeValues = new HashMap<>(); conditionExpressionAttributeValues.put(":attrValue", AttributeValue.builder().s("foo").build()); String conditionExpression = "attr = :attrValue"; // Create a DeleteExpression object to specify the delete conditionDeleteExpression deleteExpression = DeleteExpression.builder() .expressionAttributeValues(conditionExpressionAttributeValues) .conditionExpression(conditionExpression) .build(); // Create a DeleteItemRequest object to specify the item to delete and the delete conditionDeleteItemRequest deleteItemRequest = DeleteItemRequest.builder() .tableName("myTable") .key(key) .deleteExpression(deleteExpression) .build(); try { // Delete the item if the delete condition is metDeleteItemResponse deleteItemResponse = client.deleteItem(deleteItemRequest); System.out.println("Successfully deleted item with delete condition: " + conditionExpression); } catch (DynamoDBException e) { System.err.println(e.getMessage()); System.exit(1); } } }
Similar Code Examples
- Update Attribute in DynamoDB Using Java
- Delete All Items in DynamoDB Using Java
- Batch Update Using DynamoDB Mapper
- Conditional Update in DynamoDB Using Java
- Batch Delete Using DynamoDB Mapper
- Query Global Secondary Index in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Get Item Request in DynamoDB Using Java
- Update Multiple Items in DynamoDB Using Java
- Delete Record in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Batch Get in DynamoDB Using Java
- Update Expression in DynamoDB Using Java
- Batch Save Using DynamoDB Mapper
- Batch Write Using DynamoDB Mapper
Better DynamoDB experience.
Try 7-day free trial. No credit card needed.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase