Delete All Items in DynamoDB Using Java (Guide w/ Code Examples)
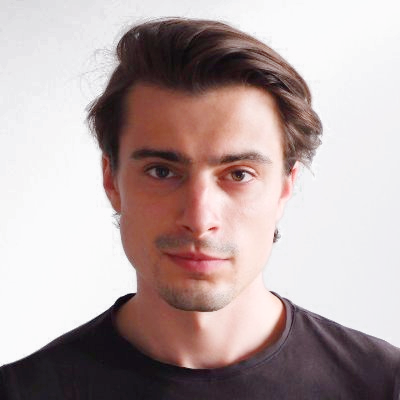
Provided by Rafal Wilinski
This Following code uses the Scan
operation to retrieve all items from the "MyTable" table, then iterates over the returned items and calls the deleteItem
method to delete each item by providing its primary key ("id" in this case). The code also uses a do-while
loop to implement pagination to retrieve all the data, even if the table is larger than 1MB.
Remember that this approach can be very slow and resource-intensive for large tables, so using the Query
operation with a specific primary key is recommended if possible, or using the Truncate
operation if you're using DynamoDB On-Demand.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.DeleteItemRequest; import com.amazonaws.services.dynamodbv2.model.ScanRequest; import com.amazonaws.services.dynamodbv2.model.ScanResult; import java.util.Map; AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); ScanRequest scanRequest = new ScanRequest().withTableName("MyTable"); ScanResult result; do { result = client.scan(scanRequest); for (Map<String, AttributeValue> item : result.getItems()) { Map<String, AttributeValue> key = new HashMap<>(); key.put("id", item.get("id")); DeleteItemRequest deleteItemRequest = new DeleteItemRequest().withTableName("MyTable").withKey(key); client.deleteItem(deleteItemRequest); } scanRequest.setExclusiveStartKey(result.getLastEvaluatedKey()); } while (result.getLastEvaluatedKey() != null);
Similar Code Examples
- Delete Expression in DynamoDB Using Java
- Get All Items from DynamoDB Using Java
- Delete Record in DynamoDB Using Java
- Query Global Secondary Index in DynamoDB Using Java
- Conditional Update in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Delete All Using DynamoDB Mapper
- Get in DynamoDB Using Java
- Batch Write Using DynamoDB Mapper
- Update Item in DynamoDB Using Java
- Batch Get in DynamoDB Using Java
- GetItem in DynamoDB Using Java
- Batch Write Item in DynamoDB Using Java
- Get Multiple Items in DynamoDB Using Java
Login to the AWS Console less. Use Dynobase.
Try 7-day free trial. No strings attached.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase