Remove Attribute in DynamoDB Using Java (Guide w/ Code Examples)
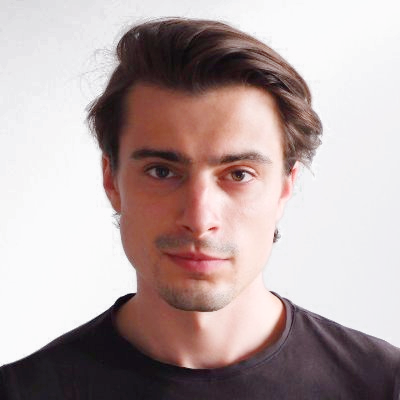
Provided by Rafal Wilinski
This example assumes that the table "myTable" has a primary key "id." This code uses the updateItem
method of the DynamoDB
class to remove an attribute named attributeName
from an item with a primary key named primaryKey
in a table named tableName
. The primary key value of the item is primaryKeyValue
. The code uses the UpdateExpression
property to specify the REMOVE
operation on the attribute to be removed, and an expressionAttributeNames
to specify the attribute name. The response is the UpdateItemResponse
object which indicates the status of the update operation.
import software.amazon.awssdk.services.dynamodb.DynamoDBClient; import software.amazon.awssdk.services.dynamodb.model.AttributeValue; import software.amazon.awssdk.services.dynamodb.model.UpdateItemRequest; import software.amazon.awssdk.services.dynamodb.model.UpdateItemResponse; import java.util.HashMap; import java.util.Map; public class RemoveAttributeExample { public static void main(String[] args) { DynamoDBClient client = DynamoDBClient.create(); String tableName = "myTable"; String primaryKey = "id"; String primaryKeyValue = "12345"; String attributeName = "attr"; Map<String, attributeValue> key = new HashMap<String, attributeValue>(); key.put(primaryKey, attributeValue.builder().s(primaryKeyValue).build()); UpdateItemRequest updateItemRequest = UpdateItemRequest.builder() .tableName(tableName) .key(key) .updateExpression("REMOVE #attributeName") .expressionAttributeNames(Map.of("#attributeName", attributeName)) .build(); UpdateItemResponse updateItemResponse = client.updateItem(updateItemRequest); System.out.println("Successfully removed attribute: " + attributeName); } }
Similar Code Examples
- Batch Insert in DynamoDB Using Java
- Batch Get in DynamoDB Using Java
- Update Multiple Attributes in DynamoDB Using Java
- Update Attribute in DynamoDB Using Java
- Delete Multiple Items in DynamoDB Using Java
- Batch Write Using DynamoDB Mapper
- Update Item in DynamoDB Using Java
- Query Local Secondary Index in DynamoDB Using Java
- Batch Delete Using DynamoDB Mapper
- Delete by Hashkey Using DynamoDB Mapper
- Get Multiple Items in DynamoDB Using Java
- Delete Record in DynamoDB Using Java
- Query Index in DynamoDB Using Java
- Batch Update Using DynamoDB Mapper
- Query Hash Key Only in DynamoDB Using Java
Login to the AWS Console less. Use Dynobase.
Try 7-day free trial. No strings attached.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase