Update Multiple Items in DynamoDB Using Java (Guide w/ Code Examples)
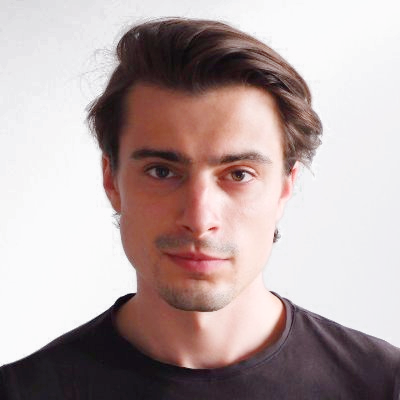
Provided by Rafal Wilinski
DynamoDB does not provide a direct method to update multiple items at once, like a "batch update" operation. However, you can use the updateItem
method in a loop to update multiple items one by one.
In the snippet shown below, a list of items to update is defined, and the updateItem
method is called for each item in the list. The updateExpression
and expressionAttributeValues
are the same for all items, but the key of the item to update is taken from the current item in the loop. This enables a batch update.
However, you can also use a Transactional Update if you wish to update multiple updates as a single operation.
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.AttributeValue.AttributeValue; import com.amazonaws.services.dynamodbv2.model.UpdateItemRequest; import java.util.List; import java.util.Map; public class MultipleUpdateExample { public static void main(String[] args) { // Create a new client AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build(); // Define a list of items to update List<Map<String, AttributeValue>> itemsToUpdate = new ArrayList<Map<String, AttributeValue>>(); itemsToUpdate.add(new HashMap<String, AttributeValue>() {{ put("id", new AttributeValue().withS("123")); }}); itemsToUpdate.add(new HashMap<String, AttributeValue>() {{ put("id", new AttributeValue().withS("456")); }}); itemsToUpdate.add(new HashMap<String, AttributeValue>() {{ put("id", new AttributeValue().withS("789")); }}); // Define the update expression String updateExpression = "SET price = :newPrice, quantity = :newQuantity"; // Define the values for the update expression Map<String, AttributeValue> expressionAttributeValues = new HashMap<String, AttributeValue>(); expressionAttributeValues.put(":newPrice", new AttributeValue().withN("999")); expressionAttributeValues.put(":newQuantity", new AttributeValue().withN("20")); for (Map<String, AttributeValue> item : itemsToUpdate) { // Create the update request UpdateItemRequest request = new UpdateItemRequest() .withTableName("Product") .withKey(item) .withUpdateExpression(updateExpression) .withExpressionAttributeValues(expressionAttributeValues); // Send the request to update the item client.updateItem(request); } } }
Similar Code Examples
- Delete Record in DynamoDB Using Java
- Batch Delete Using DynamoDB Mapper
- Delete by Hashkey Using DynamoDB Mapper
- Get All Items from DynamoDB Using Java
- Batch Update Using DynamoDB Mapper
- Batch Query in DynamoDB Using Java
- Delete Table in DynamoDB Using Java
- Query Global Secondary Index in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Delete Expression in DynamoDB Using Java
- Update Item in DynamoDB Using Java
- Get Multiple Items in DynamoDB Using Java
- Batch Insert in DynamoDB Using Java
- Remove Item in DynamoDB Using Java
- Get in DynamoDB Using Java
Better DynamoDB experience.
Try 7-day free trial. No credit card needed.
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase