DynamoDB: Query Group By (Guide w/ Code Examples)
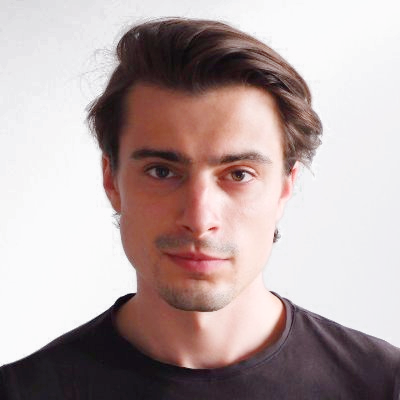
Provided by Rafal Wilinski
There is no built-in "group by" functionality in the DynamoDB query method. However, you can retrieve all the data from the table and use JavaScript to group the results by a specific attribute.
For example, you can retrieve all the items from the table, iterate through them and add them to a JavaScript object that is keyed by the attribute you want to group by.
const AWS = require('aws-sdk'); const dynamoDb = new AWS.DynamoDB.DocumentClient(); const params = { TableName: 'tableName', ProjectionExpression: 'attribute1, attribute2, attribute3', }; dynamoDb.scan(params, (error, data) => { if (error) { console.error(error); return; } const groupedData = data.Items.reduce((acc, item) => { const groupKey = item.attribute1; if (!acc[groupKey]) { acc[groupKey] = []; } acc[groupKey].push(item); return acc; }, {}); console.log(groupedData); });
This will allow you to group the data by the attribute attribute1
and you can access the data by their group key.
Please note that, depending on the size of the table and the number of items that need to be grouped, this approach may not be efficient and may consume a large amount of memory.
Similar Code Examples
- DynamoDB: Not Begins With
- DynamoDB: Delete
- DynamoDB: GetItem
- DynamoDB: Get List Of Items
- DynamoDB: Get Table
- DynamoDB: Get Last Inserted Item
- DynamoDB: Not Between
- DynamoDB: Like
- DynamoDB: Get Multiple Items
- DynamoDB: Get All Items
- DynamoDB Get in Typescript
- DynamoDB: Query Global Secondary Index in Nodejs
- DynamoDB: Get By ID
- DynamoDB: Get Unique Values
- DynamoDB: Query Count
Spend less time in the AWS console, use Dynobase.
Start your 7-day free trial today
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase