Query Date Range in DynamoDB Using Java (Guide w/ Code Examples)
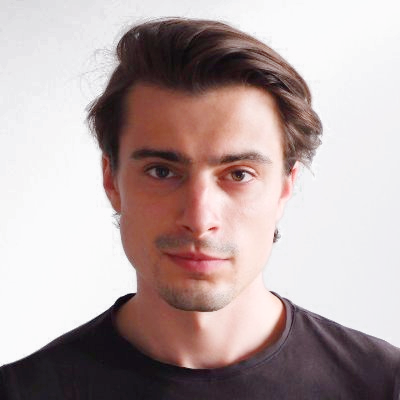
Provided by Rafal Wilinski
This example queries the MyTable
table for items where the date
attribute is between one month ago and now. The Calendar
class is used to get the current date and a date one month ago, which are then passed as parameters to the query.
import java.util.Calendar; import java.util.HashMap; import java.util.Map; import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.model.AttributeValue; import com.amazonaws.services.dynamodbv2.model.Condition; import com.amazonaws.services.dynamodbv2.model.QueryRequest; import com.amazonaws.services.dynamodbv2.model.QueryResult; public class QueryDateRangeExample { public static void main(String[] args) { AmazonDynamoDB client = AmazonDynamoDBClientBuilder.defaultClient(); Calendar calendar = Calendar.getInstance(); java.util.Date now = calendar.getTime(); calendar.add(Calendar.MONTH, -1); java.util.Date oneMonthAgo = calendar.getTime(); Map<String, AttributeValue> attributeValues = new HashMap<>(); attributeValues.put(":start", new AttributeValue().withS(oneMonthAgo.toString())); attributeValues.put(":end", new AttributeValue().withS(now.toString())); QueryRequest queryRequest = new QueryRequest() .withTableName("MyTable") .withKeyConditionExpression("date BETWEEN :start AND :end") .withExpressionAttributeValues(attributeValues); QueryResult result = client.query(queryRequest); result.getItems().forEach(item -> System.out.println(item)); } }
Similar Code Examples
- Insert in DynamoDB Using Java
- Get in DynamoDB Using Java
- Conditional Update in DynamoDB Using Java
- Delete Record in DynamoDB Using Java
- Update Item in DynamoDB Using Java
- Remove with DynamoDB Mapper
- Delete Table in DynamoDB Using Java
- Batch Insert in DynamoDB Using Java
- Batch Write Item in DynamoDB Using Java
- Query Hash Key Only in DynamoDB Using Java
- Update Multiple Items in DynamoDB Using Java
- Query Index in DynamoDB Using Java
- Batch Read in DynamoDB Using Java
- Batch Write Using DynamoDB Mapper
- Delete Expression in DynamoDB Using Java
Better DynamoDB experience.
Start your 7-day free trial today
Product Features
DynamoDB Tools
DynamoDB Info
© 2024 Dynobase