DynamoDB BatchWriteItem in Typescript (Guide w/ Code Examples)
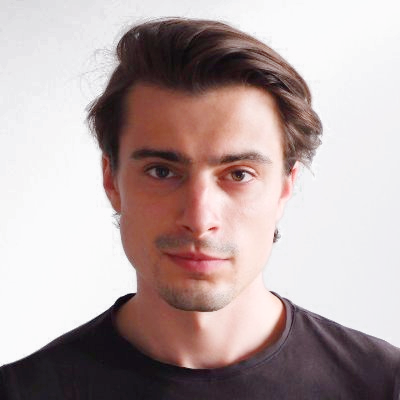
Provided by Rafal Wilinski
The batchWriteItem
method in AWS JavaScript SDK can be used to write multiple items to one or more tables in a single request. In TypeScript, you can use the aws-sdk
library to interact with DynamoDB. Here's an example of how you can use the batchWriteItem
method in a TypeScript function:
import * as AWS from 'aws-sdk'; const dynamoDb = new AWS.DynamoDB.DocumentClient(); export async function batchWriteItems(items: any[], tableName: string) { const params = { RequestItems: { [tableName]: items.map((item: any) => ({ PutRequest: { Item: item } })) } }; try { const data = await dynamoDb.batchWrite(params).promise(); console.log(data); return data; } catch (err) { console.log(err); throw new Error(err); } }
Similar Code Examples
- DynamoDB: Get Query
- DynamoDB: Attribute Not Null
- DynamoDB: Get Table
- DynamoDB: Delete Multiple Items in Javascript
- DynamoDB: Get Last Inserted Item
- DynamoDB: Get By ID
- DynamoDB: Batch Get
- DynamoDB: Query Ends With
- DynamoDB: BatchGetItem
- DynamoDB: Delete Table
- DynamoDB: Create if Not Exists
- DynamoDB: Get List Of Items
- DynamoDB: Get Multiple Items
- DynamoDB: Query Count
- DynamoDB: Get All Items
Better DynamoDB experience.
Start your 7-day free trial today
Product Features
DynamoDB Tools
DynamoDB Info
© 2025 Dynobase